Next.js 13: clean setup with TypeScript, eslint and prettier
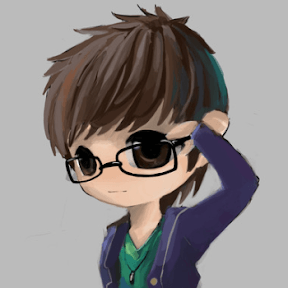
Date:
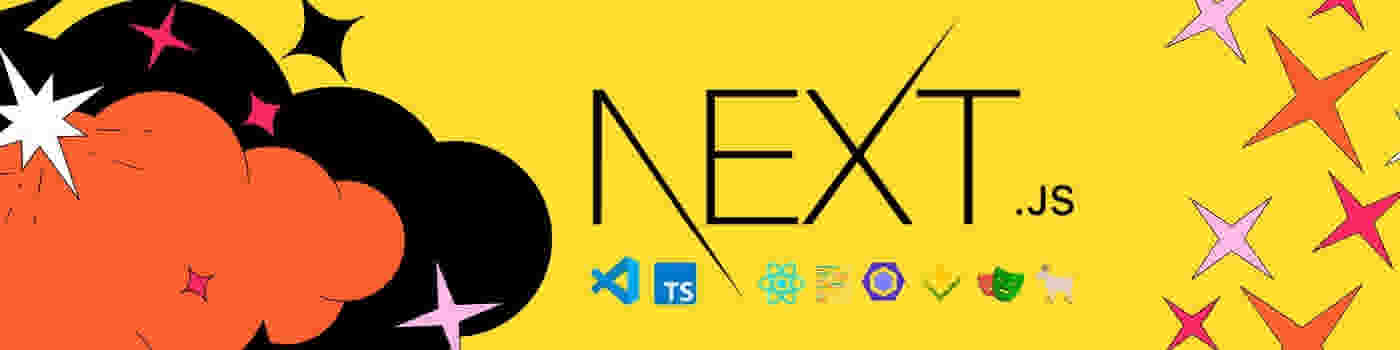
1. Introduction
In this article, we introduce to process to setup eslint and prettier for a Next.js application. You can find the source code in this repo.
2. Linting vs. Formatting
Whenever we start a new project, we setup the coding environments that we are most comfortable with: desk, chair, mouse, keyboard, monitor, IDE, theme, font, and a lot more. Usually I will setup the linting and formatting as well.
A quick but clear distinguishment between them: Linting is distinct from Formatting because linting analyzes how the code runs and detects errors whereas formatting only restructures how code appears.
3. Eslint
Following the official instructions to install Next.js, npx create-next-app@latest
setups the default eslint as { "extends": "next/core-web-vitals" }
(file .eslintrc.json
).
According to the doc:
next/core-web-vitals
updateseslint-plugin-next
to error on a number of rules that are warnings by default if they affect Core Web Vitals .
So what does eslint-plugin-next
define? Checkout the doc here, the rules defined by eslint-plugin-next
includes @next/next/google-font-display, @next/next/google-font-preconnect, @next/next/inline-script-id, etc.
If these look enough for you, stop here and jump to Prettier section below.
For me, an experienced front-end coder, I have more weapons to arm. I usually add these eslint plugins:
eslint-plugin-import: This plugin intends to support linting of ES2015+ (ES6+) import/export syntax, and prevent issues with misspelling of file paths and import names. Rules include:
import/first: This rule reports any imports that come after non-import statements.
import/newline-after-import: Enforces having one or more empty lines after the last top-level import statement or require call.
import/no-duplicates: Reports if a resolved path is imported more than once.
... ...
eslint-plugin-simple-import-sort: sorts the
import
statements in desired order.eslint-plugin-react: React specific linting rules for
eslint
eslint-plugin-react-hooks: This ESLint plugin enforces the Rules of Hooks.
If you would like to add some of plugins above, run:
Now my file .eslintrc.json
looks like:
Here we also extends the prettier
as suggested by the doc:
ESLint also contains code formatting rules, which can conflict with your existing Prettier setup. We recommend including eslint-config-prettier in your ESLint config to make ESLint and Prettier work together.
4. Prettier
prettier
does not come by default, we need to add it by yarn add -D prettier.
Then remember to add it to the extends
list in file .eslintrc.json
.If you are using tailwindcss like me, install prettier-plugin-tailwindcss to automatically sort tailwind classes with prettier:
Then add the following to your file .prettierrc.json
:
Usually I add a script to package.json to allow me to format mannually:
5. IntelliJ IDEA
We want to config IntelliJ to respect eslint and prettier. Search eslint
in the Preference
window, enable it manually:
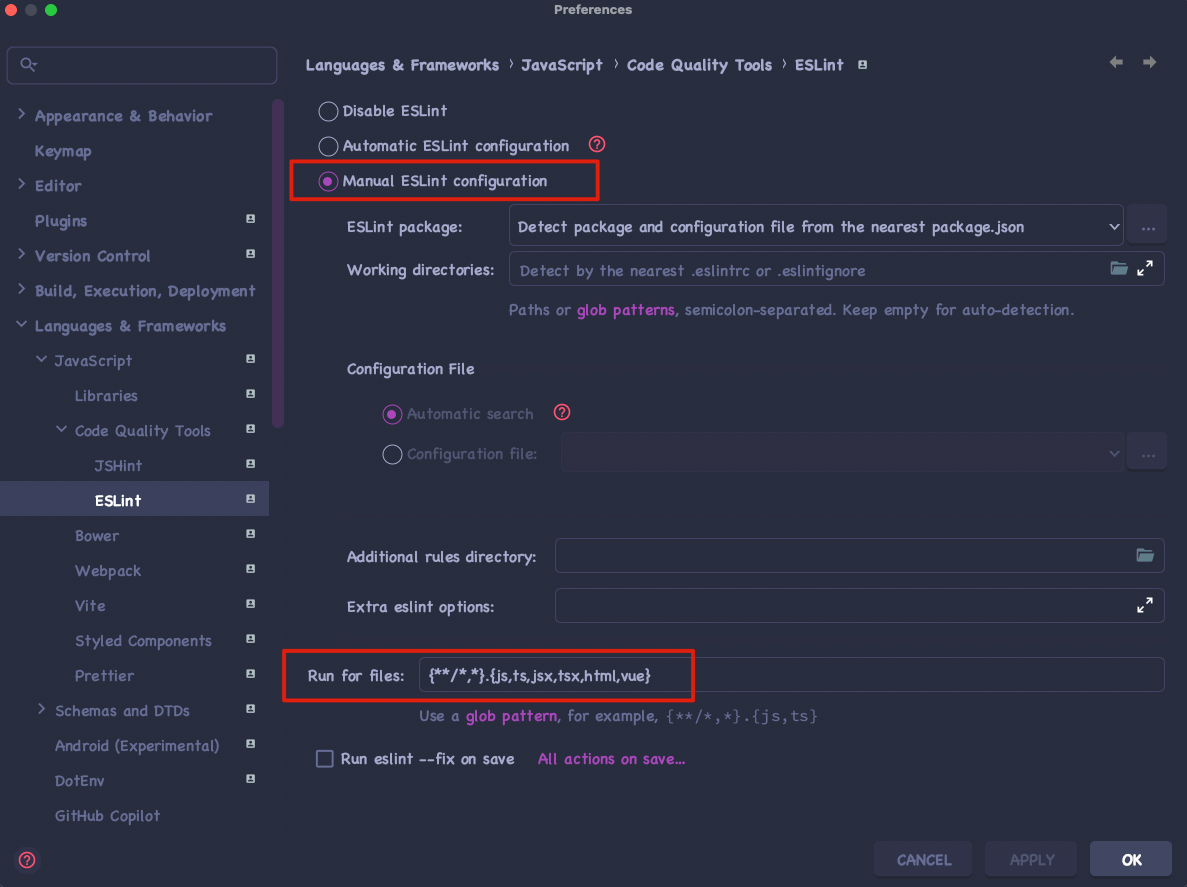
After that, whenever there is any linting errors, IntelliJ will show an error tooltip allowing you to fix it automatically. I would also usually config the Actions on Save
to do code formatting and linting:
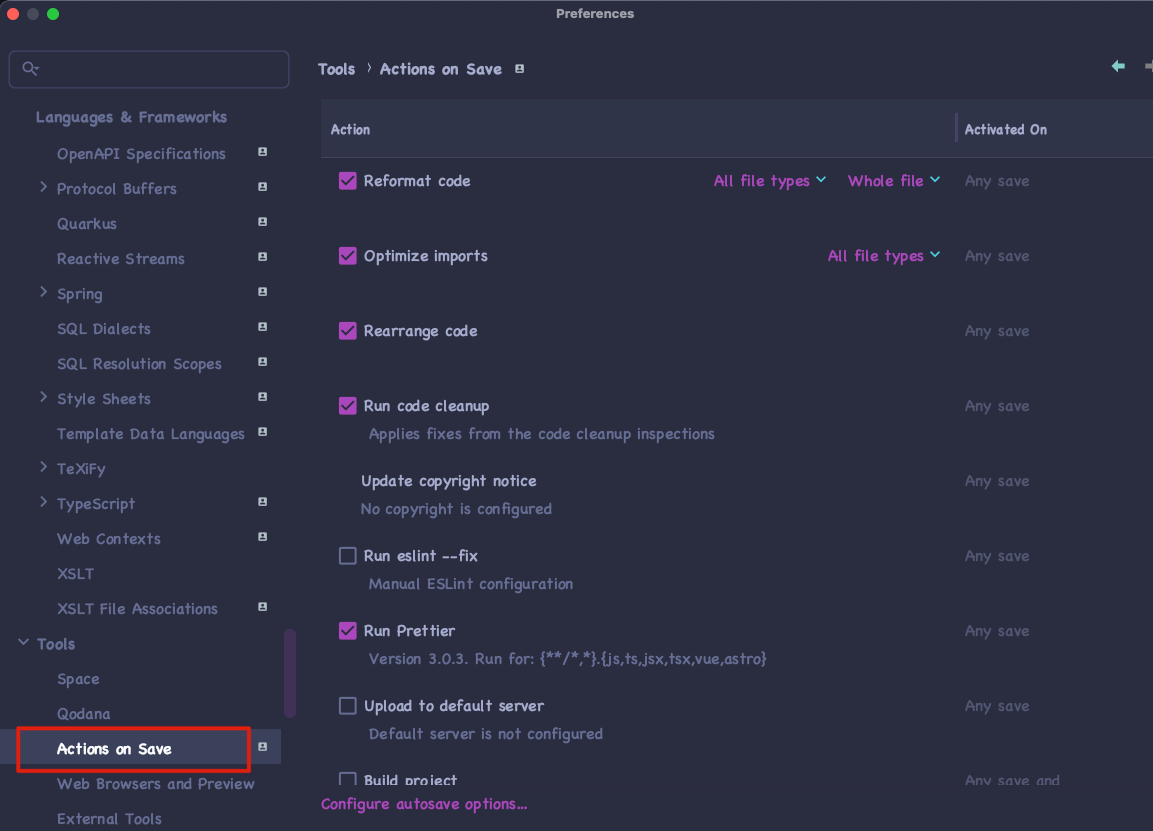
Note: you may need to reopen the project to apply the settings successfully.